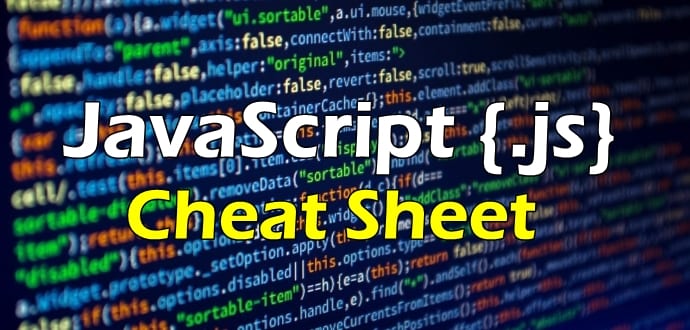
JavaScript often abbreviated as “JS”, is a high-level, dynamic, untyped, interpreted run-time language.It has been standardized in the ECMAScript language specification. Alongside HTML and CSS, JavaScript is one of the three core technologies of World Wide Web content production.
Cheat Sheet for JavaScript:
Expressions Syntax
^
|
Start of string
|
$
|
End of string
|
.
|
Any single character
|
(a|b)
|
a or b
|
(…)
|
Group section
|
[abc]
|
In range (a, b or c)
|
[^abc]
|
Not in range
|
\s
|
White space
|
a?
|
Zero or one of a
|
a*
|
Zero or more of a
|
a*?
|
Zero or more, ungreedy
|
a+
|
One or more of a
|
a+?
|
One or more, ungreedy
|
a{3}
|
Exactly 3 of a
|
a{3,}
|
3 or more of a
|
a{,6}
|
Up to 6 of a
|
a{3,6}
|
3 to 6 of a
|
a{3,6}?
|
3 to 6 of a, ungreedy
|
\
|
Escape character
|
[:punct:]
|
Any punctuation symbol
|
[:space:]
|
Any space character
|
[:blank:]
|
Space or tab
|
JavaScript Dates
Date()
|
setMonth()
|
getDate()
|
setFullYear()
|
getDay()
|
setHours()
|
getMonth
|
setMinutes()
|
getFullYear
|
setSeconds()
|
getYear
|
setMilliseconds()
|
getHours
|
setTime()
|
getMinutes
|
setUTCDate()
|
getSeconds
|
setUTCDay()
|
getMilliseconds
|
setUTCMonth()
|
getTime
|
setUTCFullYear()
|
getTimezoneOffset()
|
setUTCHours()
|
getUTCDate()
|
setUTCMinutes()
|
getUTCDay()
|
setUTCSeconds()
|
getUTCMonth()
|
setUTCMilliseconds()
|
getUTCFullYear()
|
toSource()
|
getUTCHours()
|
toString()
|
getUTCMinutes()
|
toGMTString()
|
getUTCSeconds()
|
toUTCString()
|
getUTCMilliseconds()
|
toLocaleString()
|
JavaScript Arrays
concat()
|
slice()
|
join()
|
sort()
|
length
|
splice()
|
pop()
|
toSource()
|
push()
|
toString()
|
reverse()
|
unshift()
|
shift()
|
valueOf()
|
JavaScript Strings
charAt()
|
slice()
|
charCodeAt()
|
split() x
|
concat()
|
substr()
|
fromCharCode()
|
substring()
|
indexOf()
|
toLowerCase()
|
lastIndexOf()
|
toUpperCase()
|
length
|
toLocaleLowerCase()
|
localeCompare()
|
toLocaleUpperCase()
|
match() x
|
toSource()
|
replace() x
|
valueOf()
|
JavaScript Functions
decodeURI()
|
isNaN()
|
decodeURIComponent()
|
Number()
|
encodeURI()
|
parseFloat()
|
encodeURIComponent()
|
parseInt()
|
escape()
|
String()
|
eval()
|
unescape()
|
JavaScript Booleans
toSource()
|
valueOf()
|
toString()
|
JavaScript Numbers and Maths
abs()
|
min()
|
acos()
|
NEGATIVE_INFINITY
|
asin()
|
PI
|
atan()
|
POSITIVE_INFINITY
|
atan2()
|
pow()
|
ceil()
|
random()
|
cos()
|
round()
|
E
|
sin()
|
exp()
|
sqrt()
|
floor()
|
SQRT1_2
|
LN10
|
SQRT2
|
LN2
|
tan()
|
log()
|
toSource()
|
LOG10E
|
toExponential()
|
LOG2E
|
toFixed()
|
max()
|
toPrecision()
|
MAX_VALUE
|
toString()
|
MIN_VALUE
|
valueOf()
|
JavaScript RegExp Object
compile()
|
lastParen
|
exec()
|
leftCOntext
|
global
|
multiline
|
ignoreCase
|
rightContext
|
input
|
source
|
lastIndex
|
test()
|
Incrementing /decrementing numbers
Increment | ++x or x++ |
Decrement | –x or x– |
The difference is that ++x (–x) returns the incremented (decremented) value, while x++(x–) returns the previous value of x.
No comments:
Post a Comment